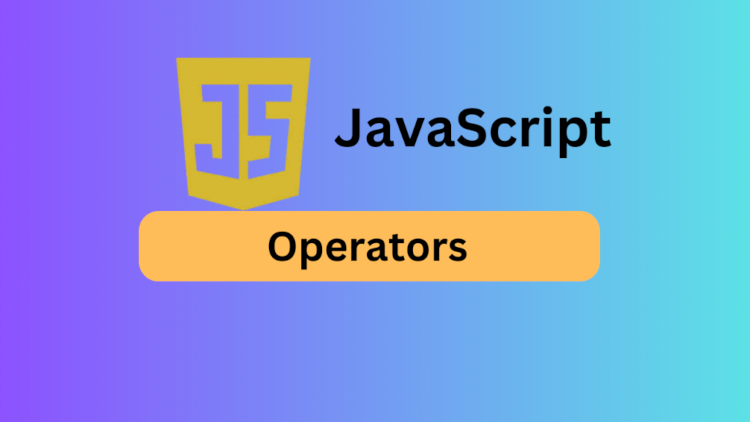
JavaScript is a powerful programming language, which is widely used for web development. Operators is very important concept, which is used to perform multiple type of operations on variables & values. In this article, we will learn about JavaScript Operators in detail with then help of examples.
What is Operators?
Operators are unique symbols in JavaScript that are used to manipulate variables and values. They give you the ability to control program logic, do computations, and work with data. JavaScript operators can be broadly classified into several categories:
1. Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations like add, subtract, multiplication, division etc.
Operator | Description | Example | Result |
+ | Addition | 6 + 6 | 12 |
- | Subtraction | 6 - 2 | 4 |
* | Multiplication | 6 * 6 | 36 |
/ | Division | 6 / 2 | 3 |
% | Modulus (Remainder) | 6 % 4 | 2 |
** | Exponentiation | 6 ** 3 | 216 |
Example
let a = 6; let b = 2; console.log(a + b); // Output: 8 console.log(a - b); // Output: 4 console.log(a * b); // Output: 12 console.log(a / b); // Output: 3 console.log(a % b); // Output: 0 console.log(a ** b); // Output: 36
2. Assignment Operators
These operators used for assign values to variables.
Operator | Description | Example | Equivalent |
= | Assigns value | x = 5 | x = 5 |
+= | Adds and assigns | x += 5 | x = x + 5 |
-= | Subtracts and assigns | x -= 5 | x = x - 5 |
*= | Multiplies and assigns | x *= 5 | x = x * 5 |
/= | Divides and assigns | x /= 5 | x = x / 5 |
%= | Modulus and assigns | x %= 5 | x = x % 5 |
**= | Exponentiation and assigns | x **= 5 | x = x ** 5 |
Example
let x = 6; x += 5; // x = 11 x /= 2; // x = 3 console.log(x);
3. Comparison Operators
These operators are used to compare two values and also return a boolean(true or false).
Operators | Description | Example | Result |
== | Equal to | 3 == '3' | true |
=== | Strict equal to | 3 === '3' | false |
!= | Not equal to | 3 != '3' | false |
!== | Strict not equal to | 3 !== '3' | true |
> | Greater than | 5 > 3 | true |
< | Less than | 5 < 3 | false |
>= | Greater than or equal to | 5 >= 5 | true |
<= | Less than or equal to | 5 <= 3 | false |
Example
console.log(5 == '5'); // true console.log(5 === '5'); // false console.log(5 > 3); // true console.log(5 <= 3); // false
Also read about JavaScript Variables
3. Logical Operators
Sometimes, we need to used multiple condition together. In this case, We use logical operators.
Operators | Description | Example | Result |
&& | Logical AND | true && false | false |
|| | Logical OR | true || false | true |
! | Logical NOT | !true | false |
Example
let x = 3, y = 5; console.log(x > 0 && y > 0); // true console.log(x > 0 || y < 0); // true console.log(!(x > 0)); // false
5. Bitwise Operators
These operators works at the binary level.
Operators | Description | Example |
& | AND | 5 & 1 |
| | OR | 5 | 1 |
^ | XOR | 5 ^ 1 |
~ | NOT | ~5 |
<< | Left Shift | 5 << 1 |
>> | Right Shift | 5 >> 1 |
Example
console.log(5 & 1); // Output: 1 console.log(5 | 1); // Output: 5 console.log(5 ^ 1); // Output: 4 console.log(~5); // Output: -6 console.log(5 << 1); // Output: 10 console.log(5 >> 1); // Output: 2
6. Ternary Operator
It is short form of if-else statement.
Example
let age = 17; let vote_status = (age >= 18) ? 'Eligible for voting' : 'Not eligible for voting'; console.log(vote_status); // Output: Not eligible for voting
7. Type Operators
These type of operators are use to check and convert data types.
Operators | Description | Example |
typeof | Returns the type | typeof 'Hello' |
instanceof | Checks if an object is an instance of a class | x instanceof Array |
Example
console.log(typeof 25); // Output: number console.log(typeof 'Welcome'); // Output: string console.log([3, 8, 11] instanceof Array); // Output: true
Conclusion
Writing effective and tidy code requires an understanding of JavaScript operators. Operators are essential to JavaScript programming because they can be used for arithmetic operations and logical decision-making. Gaining proficiency with these operators will enable you to develop better scripts and efficiently optimize your code.