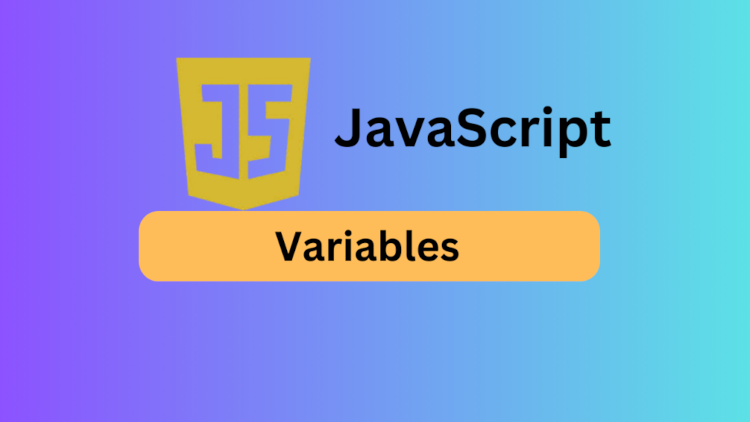
Variables are one of the most fundamental concepts in any language. Every language is used variables to store different types of values. Those variables are used in entire program and also can change according to need. In JavaScript, we declare variables by several ways & each variables have own behavior and scopes. In this article, we will learn about JavaScript Variables, its uses & scopes in depth with the help of examples.
Declaring Variables
JavaScript provides three ways to declare variables:
- Using var
- Using let
- Using const
Using var
We use var to declare variable in JavaScript before ES6 (2015). At that time, it was only a single way to define variables.
Example
var name = "Alice"; console.log(name);
Output
Alice
Characteristics of var
It accessible within the function where it was defined. It can also be re-declare.
Example
var age = 25; var age = 30; console.log(age);
Output
30
console.log(a); var a = 5; console.log(a);
Output
undefined
5
Here we can use variable without declare. It will never give error.
Using let
Let was first used in ES6 and is recommended for variables that fluctuate over time.
Example
let age = 25; age = 30; console.log(age);
Output
30
Characteristics of let
It is only accessible within the {} block in which it is defined. It cannot be re-declared in the same scope.
Example
let x = 10; let x = 20;
Output
SyntaxError: Identifier 'x' has already been declared
console.log(a); let a = 5; console.log(a);
Output
ReferenceError: Cannot access 'a' before initialization
Using const
When the value of the variable should never be changed, use const.
Example
const PI = 3.14; console.log(PI);
Output
3.14
Characteristics of const
It is only accessible within the {} block in which it is defined. It cannot be reassigned.
Example
const city = "New York"; city = "Los Angeles";
Output
TypeError: Assignment to constant variable.
Doesn’t make objects immutable: The reference alone, not the actual object, is unchangeable.
const person = { name: "Alice" }; person.name = "Bob"; console.log(person.name);
Output
Bob
Also read about Inheritance in PHP
Variable Scope
The scope of your code establishes where a variable can be accessed.
Global Scope
A globally scoped variable is one that is declared outside of any function.
Example
var globalVar = "I'm global!"; console.log(globalVar);
Output
I'm global!
It will be accessible everywhere.
Function Scope
A variable that is declared inside a function can only be accessed within that function.
Example
function greet() { var message = "Hello"; console.log(message); } console.log(message);
Output
ReferenceError: message is not defined
This error comes because message variable declared under function.
Block Scope
Block-scoped variables are those that are declared using let or const enclosed in {}.
Example
{ let blockVar = "Inside block"; } console.log(blockVar);
Output
ReferenceError: blockVar is not defined
Variable Naming Rules
- It can include letters, numbers, _, and $.
- It must start with a letter, _, or $.
- It cannot use JavaScript reserved keywords like let, private, return short etc.
- JavaScript variables are case-sensitive, It means userName and username are different.
Valid names examples
let myVar; let _myVar; let $price;
Invalid names examples
let 123var; let let;
Data Types in Variables
JavaScript variables are capable of storing several kinds of data.
Data Type | Type | Description |
String | Primitive | Text enclosed in quotes |
Number | Primitive | Integer or floating-point |
Boolean | Primitive | true or false |
Symbol | Primitive | Unique identifiers |
Object | Non-Primitive | A collection of key-value pairs |
Array | Non-Primitive | Ordered list of values |
Function | Non-Primitive | Reusable blocks of code |
Example
let name = "Alice"; //String Type let score = 100; //Number Type let price = 99.99; //Number Type let isActive = true; //Boolean Type let sym = Symbol("description"); //Symbol Type let person = { name: "Alice", age: 30 }; //Object Type let colors = ["red", "blue", "green"]; //Array type //Function Type function greet() { return "Hello!"; }
Conclusion
A fundamental idea that controls data manipulation and storage is JavaScript variables. To improve scoping and steer clear of frequent errors, let and const are advised. You can build more effective and error-free JavaScript code by being aware of scopes, data types, hoisting, and best practices.