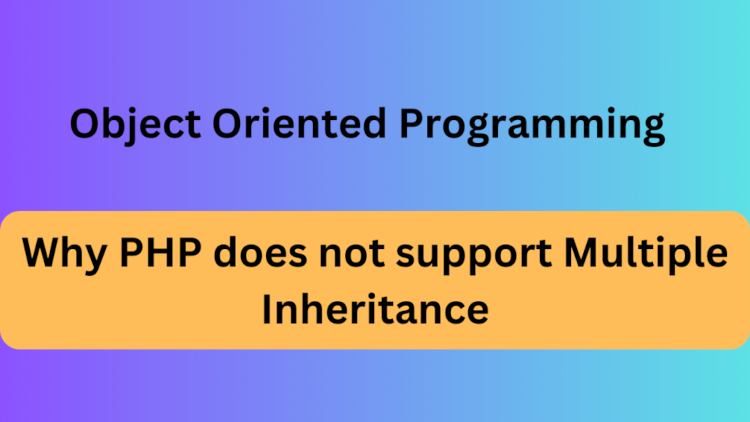
Inheritance are a very important part in PHP object-oriented programming (OOP). In this article, we will learn about Why PHP does not support Multiple Inheritance in detail with help of examples.
PHP doesn't allow multiple inheritance since it can bring ambiguity and complexity in object-oriented programming. Multiple inheritance is when a class directly inherits from numerous parent classes.
Detailed Explanation
- Indecision and the Diamond Issue
The diamond problem is the main problem with multiple inheritance. It occurs when a child class inherits from two parent classes that have the same method or property name.
Example
<?php class A { public function Hello() { echo "Hello from A\n"; } } class B { public function Hello() { echo "Hello from B\n"; } } // Hypothetical case: A class inheriting from both A and B class C extends A, B { // Conflict: Which "Hello()" method should C inherit? A's or B's? } $c = new C(); $c->Hello(); // Ambiguity: Should this call A's or B's Hello() method? ?>
There is uncertainty and the possibility of errors because there is no explicit rule on which method should be inherited in these situations.
2. Increased Complexity
The language and the resulting code become more complex due to multiple inheritance. Multiple parent classes may cause behavior or property conflicts that make it harder for developers to understand and maintain the code.
3. Promoting Alternative Solutions and Simplicity
Multiple inheritance is frequently superfluous when there are other options available, and PHP places a high value on design simplicity. PHP avoids the problems of multiple inheritance by using alternative mechanisms that offer the same functionality.
Also read about Inheritance in PHP
Multiple Inheritance Alternatives
Traits
PHP has a feature called traits that lets you reuse methods in different classes. Classes can be "injected" with traits, which function similarly to reusable building components.
<?php trait Logger { public function log($message) { echo "Log: $message\n"; } } trait Notifier { public function notify($message) { echo "Notify: $message\n"; } } class User { use Logger, Notifier; // Include both traits } $user = new User(); $user->log("This is a log message."); // Log: This is a log message. $user->notify("This is a notification."); // Notify: This is a notification. ?>
Advantages of Traits
- No ambiguity: The class is explicitly updated to include methods from characteristics.
- Reusability without inheritance.
Interfaces
Interfaces give flexibility and prevent the diamond problem by enabling a class to implement several contracts.
Example
<?php interface Logger { public function log($message); } interface Notifier { public function notify($message); } class User implements Logger, Notifier { public function log($message) { echo "Log: $message\n"; } public function notify($message) { echo "Notify: $message\n"; } } $user = new User(); $user->log("Logging message."); $user->notify("Notifying message."); ?>
Advantages of Interfaces
- Enforces method implementation in the child class.
- Permits the implementation of multiple interfaces by a class, simulating multiple inheritance.
Conclusion
Multiple inheritance is avoided by PHP in order to preserve simplicity and avoid ambiguity brought on by method/property conflicts (such as the diamond problem). Rather, PHP offers composition, interfaces, and traits as more organized and clean substitutes to accomplish the functionality of multiple inheritance without its disadvantages. These tools improve the modularity, reusability, and maintainability of code.